Xamarin Forms – Using OnPlatform
While developing Xamarin Forms applications you will often find yourself in a situation where you need to set different values for a certain property depending on the operating system.
OnPlatform allows you to do just that and can be used both from C# code and XAML. Let’s look at a few examples. For this article, we’ll be working with a new master-detail project.
Using OnPlatform with XAML
In the about page there’s a Learn mode button, let’s make it’s color platform dependent: green for Android, orange for iOS and purple for UWP.
<Button Margin="0,10,0,0" Text="Learn more"
BackgroundColor="{OnPlatform Android=Green, iOS=Orange, UWP=Purple}"
Command="{Binding OpenWebCommand}"
TextColor="White" />
Code language: HTML, XML (xml)
And let’s look at the result:
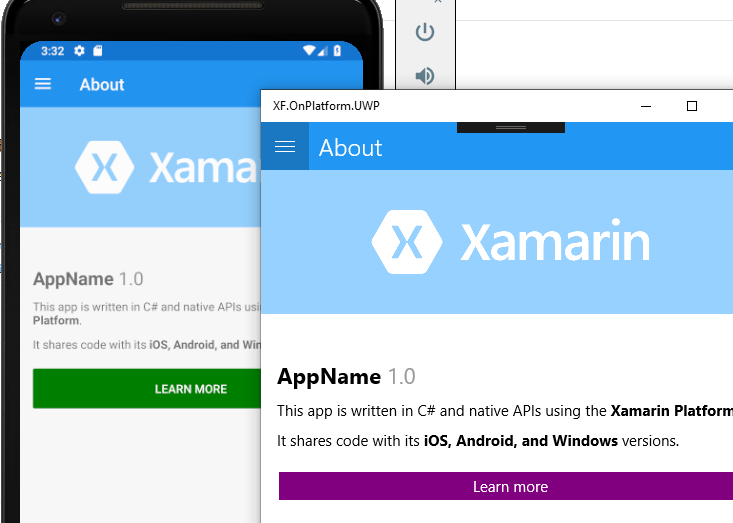
Alternatively, you could also use the following syntax which is more convenient when dealing with fancy-er data types.
<Button Margin="0,10,0,0" Text="Learn more"
Command="{Binding OpenWebCommand}"
TextColor="White">
<Button.BackgroundColor>
<OnPlatform x:TypeArguments="Color">
<On Platform="Android" Value="Green"/>
<On Platform="iOS" Value="Orange"/>
<On Platform="UWP" Value="Purple"/>
</OnPlatform>
</Button.BackgroundColor>
</Button>
Code language: HTML, XML (xml)
Using OnPlatform with C# (deprecated)
Same requirements as above, but this time from C# instead of XAML. First we’ll give our button a x:Name=”LearnMoreButton” and then in the code behind we’ll write the following:
Device.OnPlatform(
Android: () => this.LearnMoreButton.BackgroundColor = Color.Green,
iOS: () => this.LearnMoreButton.BackgroundColor = Color.Orange,
WinPhone: () => this.LearnMoreButton.BackgroundColor = Color.Purple,
Default: () => this.LearnMoreButton.BackgroundColor = Color.Black);
Code language: C# (cs)
Same result as before. WinPhone maps to UWP and you also get to specigy a default value for the rest of the platforms. This method is deprecated as of XF 2.3.4, and it’s recommended you write your own switch case on Device.RuntimePlatform instead.
Using Device.RuntimePlatform instead
The code above can be translated to:
switch (Device.RuntimePlatform)
{
case Device.Android:
LearnMoreButtonSwitch.BackgroundColor = Color.Green;
break;
case Device.iOS:
LearnMoreButtonSwitch.BackgroundColor = Color.Orange;
break;
case Device.UWP:
LearnMoreButtonSwitch.BackgroundColor = Color.Purple;
break;
default:
LearnMoreButtonSwitch.BackgroundColor = Color.Black;
break;
}
Code language: C# (cs)
The supported platform values currently are: iOS, Android, UWP, macOS, GTX, Tizen and WPF.
As usual, you can find the sample project source code on GitHub.